シングルトンを複数の .xib ファイルで使用する
Singleton クラスのプロパティを 複数の InterfaceBuilder(.xib) ファイルから参照できるようにすれば、同期のためのソースコードを簡略することができます。
各 .xib ファイルで同一の Singleton インスタンスが得られるように、SelectionManager という Singleton を定義します。 ここで、allocWithZone: メソッドは常に singleton インスタンスを返すようにしてください。
// SelectionManager.h #import <Cocoa/Cocoa.h> @interface SelectionManager : NSObject { @private id selection_; } @property (assign, readwrite) id selection; + (id)sharedInstance; @end
// SelectionManager.m #import "SelectionManager.h" @interface SelectionManager (Singleton) static id singleton = nil; static BOOL willDelete = NO; @end @implementation SelectionManager @synthesize selection = selection_; + (id)sharedInstance { @synchronized(self) { if (!singleton) { singleton = [[[self class] alloc] init]; } } return singleton; } #pragma mark Singleton Methods // 1つ目のインスタンスは作成し,それ以降のインスタンスは作成しない. + (id)allocWithZone:(NSZone *)zone { @synchronized(self) { if (!singleton) { singleton = [super allocWithZone:zone]; } } return singleton; // 複数の .xib ファイルで同一のインスタンスを扱う } // オブジェクトのコピーを阻止. - (id)copyWithZone:(NSZone *)zone { return self; } // Singletonインスタンスを削除するためのクラスメソッド. + (void)deleteInstance { if (singleton) { @synchronized(self) { willDelete = YES; [singleton release]; singleton = nil; willDelete = NO; } } } - (id)retain { return self; } - (NSUInteger)retainCount { return UINT_MAX; } - (void)release { @synchronized(self) { if (willDelete) { [super release]; } } } - (id)autorelease { return self; } @end
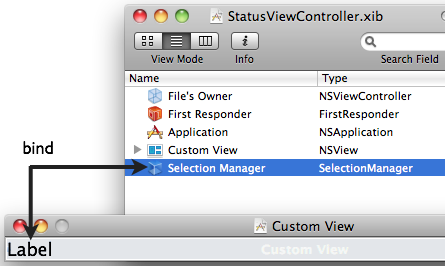
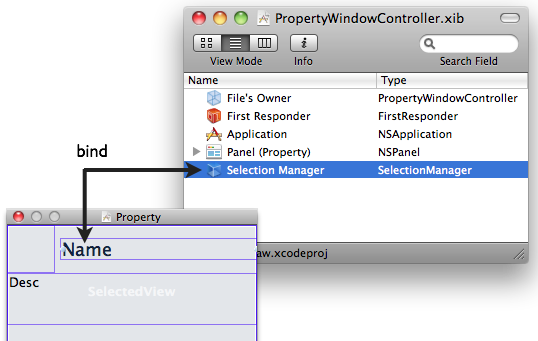
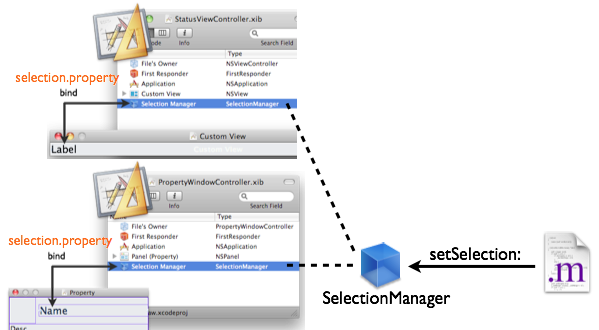
これで、SelectionManager の selection プロパティが setSelection: メソッドで変更された時、自動的にバインディングしている箇所の値が更新されます。

- 作者: 木下誠
- 出版社/メーカー: ビー・エヌ・エヌ新社
- 発売日: 2009/03/27
- メディア: 単行本(ソフトカバー)
- 購入: 9人 クリック: 119回
- この商品を含むブログ (30件) を見る

Cocoa Design Patterns (Developer's Library)
- 作者: Erik M. Yacktman, Donald A. Buck
- 出版社/メーカー: Addison-Wesley Professional
- 発売日: 2009/09/01
- メディア: ペーパーバック
- 購入: 1人 クリック: 6回
- この商品を含むブログ (3件) を見る